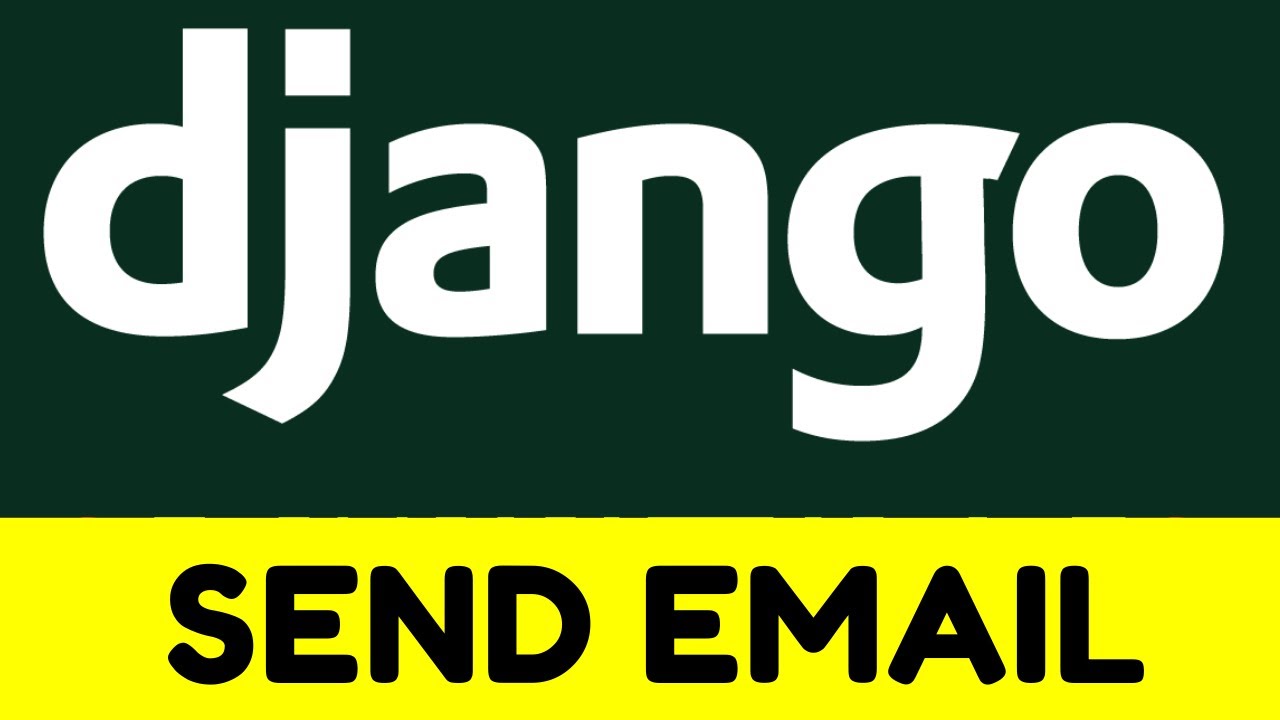
Sending Simple E-Mails using Django
Introduction
Django comes with a ready and easy-to-use light engine to send e-mail. Similar to Python you just need an import of smtplib. In Django you just need to import django.core.mail.
To Start sending an E-Mail: Edit the setting.py file
Procedures to Send Simple E-mail
Let's create a "sendSimpleEmail" view to send a simple e-mail.
from django.shortcuts import renderfrom .forms import EmailFormfrom django.core.mail import send_mailfrom django.conf import settingsdef sendSimpleEmail(request):# create a variable to keep track of the formmessageSent = False# check if form has been submittedifrequest.method == 'POST':form = EmailForm(request.POST)# check if data from the form is cleanifform.is_valid():cd = form.cleaned_datasubject = "Sending an email with Django"message = cd['message']# send the email to the recipentsend_mail(subject, message,settings.DEFAULT_FROM_EMAIL, [cd['recipient']])# set the variable initially created to TruemessageSent = Trueelse:form = EmailForm()returnrender(request, 'index.html', {'form': form,'messageSent': messageSent,})
The details of the parameters of send_mail −
- subject − E-mail subject.
- message − E-mail body.
- from_email − E-mail from.
- recipient_list − List of receivers’ e-mail address.
- fail_silently − Bool, if false send_mail will raise an exception in case of error.
- auth_user − User login if not set in settings.py.
- auth_password − User password if not set in settings.py.
- connection − E-mail backend.
- html_message − The e-mail will be multipart/alternative.
from django.conf.urls import patterns, url urlpatterns = paterns('myapp.views', url(r'^simpleemail/(?P<emailto>[\w.%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,4})/', 'sendSimpleEmail' , name = 'sendSimpleEmail'),)
So when accessing /myapp/simpleemail/saisrivatsau1998@gmail.com, The output the page shows with the count of mail sent.
Then we can see the output with One messages were sent successfully.
Sending Multiple Mails with send_mass_mail
The method returns the number of messages successfully delivered. This is same as send_mail but takes an extra parameter; data tuple, our sendMassEmail view will then be −
from django.core.mail import send_mass_mail from django.http import HttpResponse def sendMassEmail(request,emailto): msg1 = ('subject 1', 'message 1', 'polo@polo.com', [emailto1]) msg2 = ('subject 2', 'message 2', 'polo@polo.com', [emailto2]) res = send_mass_mail((msg1, msg2), fail_silently = False) return HttpResponse('%s'%res)
Let's create a URL to access our views:-
from django.conf.urls import patterns, url urlpatterns = paterns('myapp.views', url(r'^massEmail/(?P<emailto1> [\w.%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,4})/(?P<emailto2> [\w.%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,4})', 'sendMassEmail' , name = 'sendMassEmail'),)
So when accessing /myapp/simpleemail/saisrivatsau1998@gmail.com, The output the page shows with the count of mail sent.
send_mass_mail parameters details are −
- data tuples − A tuple where each element is like (subject, message, from_email, recipient_list).
- fail_silently − Bool, if false send_mail will raise an exception in case of error.
- auth_user − User login if not set in settings.py.
- auth_password − User password if not set in settings.py.
- connection − E-mail backend.
Then we can see the output with two messages were sent successfully.